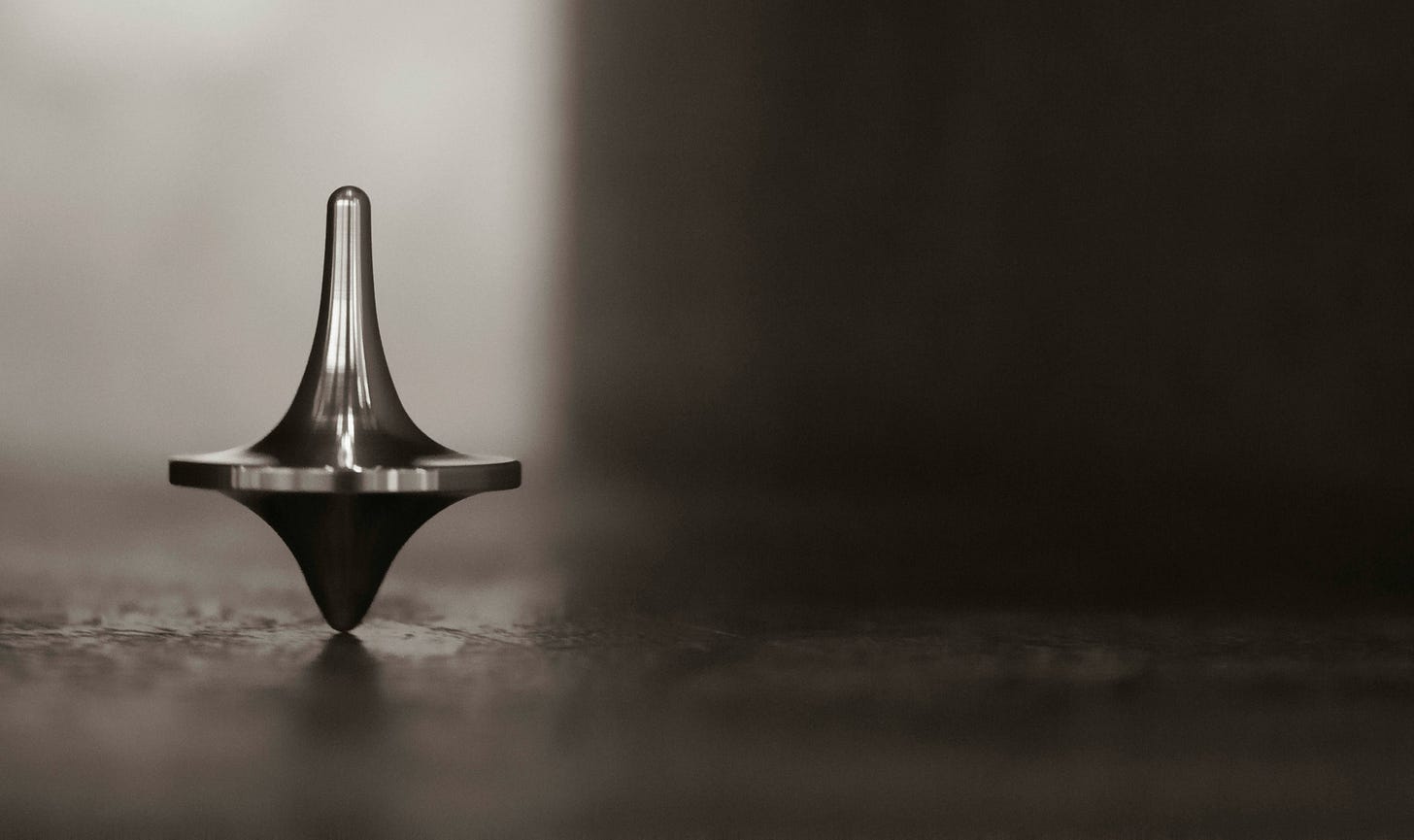
We’re building Athena to be a best in class VM and we’re studying other projects and ecosystems to ensure this. But what does best in class actually mean? The reality is if you ask ten different people you’ll get ten different answers, and everything is a tradeoff. Designing a best in class system is a balancing act since by definition you can’t have everything. Here are three goals of the project that are the most important to me, and some of the tradeoffs among them.
Thing #1: Developer Friendly 👨💻
One of the biggest, most important goals for Athena is that it have a best in class developer experience. What does that mean? We could talk about programming language and tools, we could talk about compiling, debugging, optimizing, and deploying, but actually the best, most concise answer is that it should be fun to develop applications on Athena.
I remember the first time I experienced the joy of programming. I remember the feeling of excitement and awe when I first saw my code successfully running, almost definitely something written in Basic that I’d copied out of a magazine! I remember feeling something similar the first time I successfully wrote networking code, the first time I wrote a CGI script, the first time I successfully designed a game world, the first time I got a game running on my TI-86 in high school. More recently I remember the first time I saw my app running on a real mobile phone, my first serverless application, my first smart contract, and of course seeing the Spacemesh network go live last year. Writing and running programs on Athena should feel like this. It should invoke a feeling of wonder and joy, and if it doesn’t, I will feel that I’ve failed in its design and construction. That’s the north star with respect to developer experience.
To speak in slightly more concrete terms Athena code should be easy to read and write. When I say “easy” I don’t mean that anyone should be able to do so without training. Rust code isn’t easy to read and it’s even harder to write, but once you get the hang of it, it’s a joy to both read and write. It’s clean and well-structured, it’s concise and information-dense, and when you get used to it, it makes a lot of sense. That’s what I mean by “easy”: that when you know what you’re doing, you can figure out what’s going on quickly and you can express the ideas in your head quickly and cleanly, and in such a way that others can read your code and understand it without too much trouble. We’re still figuring out the details but I believe it’ll be possible to program for Athena in Rust without too many modifications.
This is a big reason we decided against EVM, Move, and Cairo. Each of those ecosystems has a lot going for it and I can say many nice things about each of them, but each requires learning a special-purpose programming language and toolchain that literally can’t be used for anything else. Move and Cairo are very exciting projects and the languages are heavily inspired by Rust—but they’re not Rust. Solana uses a variant of Rust but not mainline Rust. We intend to use plain old Rust thanks to RISC-V and its LLVM support (more on this in a moment), something that wasn’t possible even a year or two ago.
The programming language is the most important tool but it’s not the entire toolchain. I’ve spent a lot of time studying developer tools inside and outside the blockchain ecosystem and from my own experience writing and deploying blockchain applications I have some idea what makes a good toolchain. The most important thing developer tools should do is automate some of the drudgery involved in programming such as removing boilerplate code and making it dead simple to compile, test, and deploy an application so that the developer can stay focused on the core business logic. I envision an Athena CLI tool that plugs directly into the Rust and LLVM toolchain and that allows applications to be tested, compiled, and deployed to testnet in seconds. It shouldn’t require complex deploy scripts and shouldn’t require the developer to think too much about accounts and signers.
The ability to simulate a running testnet locally is also critical. Different projects take different approaches here but broadly speaking we want something like the Bitcoin regtest network: you mine blocks as fast or as slowly as you like and simulate a real running network. In a chain like Spacemesh with a relatively slow block time this is doubly important.
Athena also needs a best in class chain explorer, something that became very clear when I began working in the Solana and Move ecosystems a few weeks ago. We may not have the resources to build all of this ourselves so we’ll rely on the community for support. With these basic pieces in place, and with familiar Rust tooling, I’m sure it’ll be a joy to write and deploy code to Athena.
Thing #2: ZK Friendly 🫥
If DevEx were our only goal we’d pick Rust and be done with it, but of course it’s not so simple! While Athena is a general-purpose, performant, secure VM it’s also intended to be natively compatible with zero knowledge proofs (ZK). As far as I’m aware it’s the first project to have both of these goals, i.e., to be performant both when run natively and when proven using ZK.
There are several ways to achieve ZK friendliness, otherwise known as succinctness. The approach taken by most zkVM projects to date is to hand code a custom circuit for the VM and to manually implement each instruction. This is a laborious, error-prone task and it’s not for the feint of heart. Nevertheless there are several zkEVM implementations that have chosen this path and there are attempts to do the same for other VMs like Move and Wasm.
Another approach is to start with the circuit and build the VM and language around it, an approach taken by projects including Cairo and Aleo. Cairo has come a long way and now looks a lot like Rust or Move but it’s still a language and a VM designed around a ZK circuit which means that certain tradeoffs and sacrifices must be made. Such a system will never be as developer friendly or as performant (in the non-ZK case) as something like actual Rust.
Very recently a third alternative has emerged. Several projects including RiscZero and SP1 have manually created a custom ZK circuit to prove the low-level RISC-V instruction set, which is a real game-changer. Thanks to these projects one can now compile a blockchain VM interpreter to RISC-V and run the entire thing inside the zkVM. While this gets the job done, and is surprisingly already almost performant and cheap enough for production, it will always be several orders of magnitude slower than running RISC-V program code directly inside the zkVM (which will in turn always be slower than running the same code natively).
It’s this final approach that we’re planning for Athena: running VM program code directly and “natively” inside the RISC-V ZK circuit. It’s an ambitious plan and to the best of my knowledge it’s never even been attempted before. There are many zkVMs and also many RISC-V VMs but no single VM that can natively target both. There’s no fundamental reason it won’t work, but it’ll require a lot of clever engineering. RISC-V VMs usually use a modified version of RISC-V code that wouldn’t work natively in a RISC-V zkVM. Even if we stay as close as possible to the RISC-V standard there will still be challenges around things like how we handle syscalls and IO, proving state reads and writes, and handling cross-contract calls.
The challenge of course is not how to make Athena ZK friendly but rather how to ensure that the other goals of the project including developer friendliness and performance don’t suffer too much as a result. With respect to developer friendliness we’ll try to make sure that Athena code looks and feels as much like normal Rust as possible. Of course there are some restrictions that every deterministic blockchain VM must impose such as allowing access only to deterministic pseudo-randomness and removing floating point math entirely or else implementing it in software. The best case scenario will be a lightweight SDK with a few blockchain-native types (accounts, addresses, programs), a little glue to handle IO and exposing functions via an ABI, and not much else. We may or may not be able to handle support for the Rust standard library.
And as for performance—see the next thing.
Thing #3: Performant 🏎️💨
I used to get excited when projects announced faster and faster VMs. We now live in an age where projects often claim upwards of 10k TPS, sometimes even 100k TPS or more (even though these numbers are always theoretical and in practice they rarely deliver even 10% of this, and also TPS is a dumb vanity metric). And these days I roll my eyes when I read numbers like these because more than anything they demonstrate ignorance towards how blockchains actually work in the wild.
The thing most people fail to understand is that the VM itself is not the bottleneck. Take EVM. You could create a highly optimized EVM implementation. You could run it on a GPU and parallelize the heck out of it and achieve 100x or even 1000x better performance in the lab. But you won’t get anywhere near that performance in situ in a real, live blockchain. For one thing EVM transactions are simply not parallelizable. Ethereum was designed to be a single-threaded state machine. Projects like Solana and Aptos have made some progress on parallelization and there are attempts to do the same with EVM which may someday succeed, but you can’t parallelize EVM transactions in production for the simple reason that each transaction depends on the transaction before it.
Even if you could hypothetically parallelize EVM transactions and achieve more throughput you’d immediately run into the next bottleneck, which is state and IO. Largely due to the PMT design it takes a huge amount of disk IO to read and write Ethereum state. You simply can’t squeeze much more performance out of modern hardware without drastically raising the requirements for running an Ethereum node. And if you solve this you’ll run into the next bottleneck, maybe network bandwidth. (This idea in computer science is called Amdahl’s Law.)
The point is that people tend to think about VM performance in isolation, i.e., in stupid, narrow-minded ways. A VM is basically a pipeline with many stages and its overall performance is only as good as its weakest link. Our goal is for Athena to be performant in all the ways that matter, not in this or that narrow way.
Having said that, I’m not that worried about performance today for three reasons. The first is that Spacemesh doesn’t have much transaction volume today so we have plenty of time to improve performance. The second I already mentioned, above: we’re building on core primitives including RISC-V that can be as performant as we need them to be. There’s no reason we can’t make Athena crazy fast when the time comes. The third is that I don’t believe in premature optimization. We’ll build Athena incrementally and scale it as needed. We’re planning ahead and making sure that Athena has everything it needs in order to scale, to support things like parallelization, and to work with really fast blockchains.
Making Athena performant in the native case will be relatively easy. As long as Athena conforms to and is able to target RISC-V without a high compile time burden—and I expect this to be the case—then it will run well natively whether we choose JIT or AOT compilation. We’ll probably start with a JIT compiler and maybe fall back to an interpreter as necessary, e.g., on platforms that don’t support compilation. We’ll design the higher-level architecture such as the account model and state in a performant fashion as well, learning from the mistakes of Ethereum and other projects.
Making Athena performant in the ZK case will be harder but we can rely on the amazing work done by projects like RiscZero, SP1, and Jolt. It wasn’t previously possible to create a VM with two execution paths, native and ZK, and to have a ZK-friendly VM that also runs at near native speed. But this is now possible which means we can avoid many of the performance tradeoffs traditionally associated with ZK friendliness. While Athena code will run more slowly inside the zkVM, because it’ll natively target the instruction set supported by these tools proving them in ZK should be extremely fast, among the fastest zkVMs. (We’ll provide benchmarks as soon as we’re able.)
There aren’t many tradeoffs between developer friendliness and performance. One possible tradeoff is compilation time: it can take a bit longer to compile a program in such a way that it’s optimized and runs as fast as possible. But we can make sure this step happens off-chain and doesn’t cost gas or cause problems for Athena executors.
In short, with Athena we want to have our cake and eat it too. It sounds too good to be true—a full-featured VM that lets you write programs in ordinary Rust, that is fully compatible with the Rust, LLVM, and RISC-V toolchain, that allows you to run programs at near-native speed inside a blockchain VM and also that also offers best in class ZK proving time. But based on our research these things are all possible today and there’s little to no theory separating us from achieving these goals, just hard engineering. We’re working on it and hope to have a proof of concept working soon. Stay tuned for more.